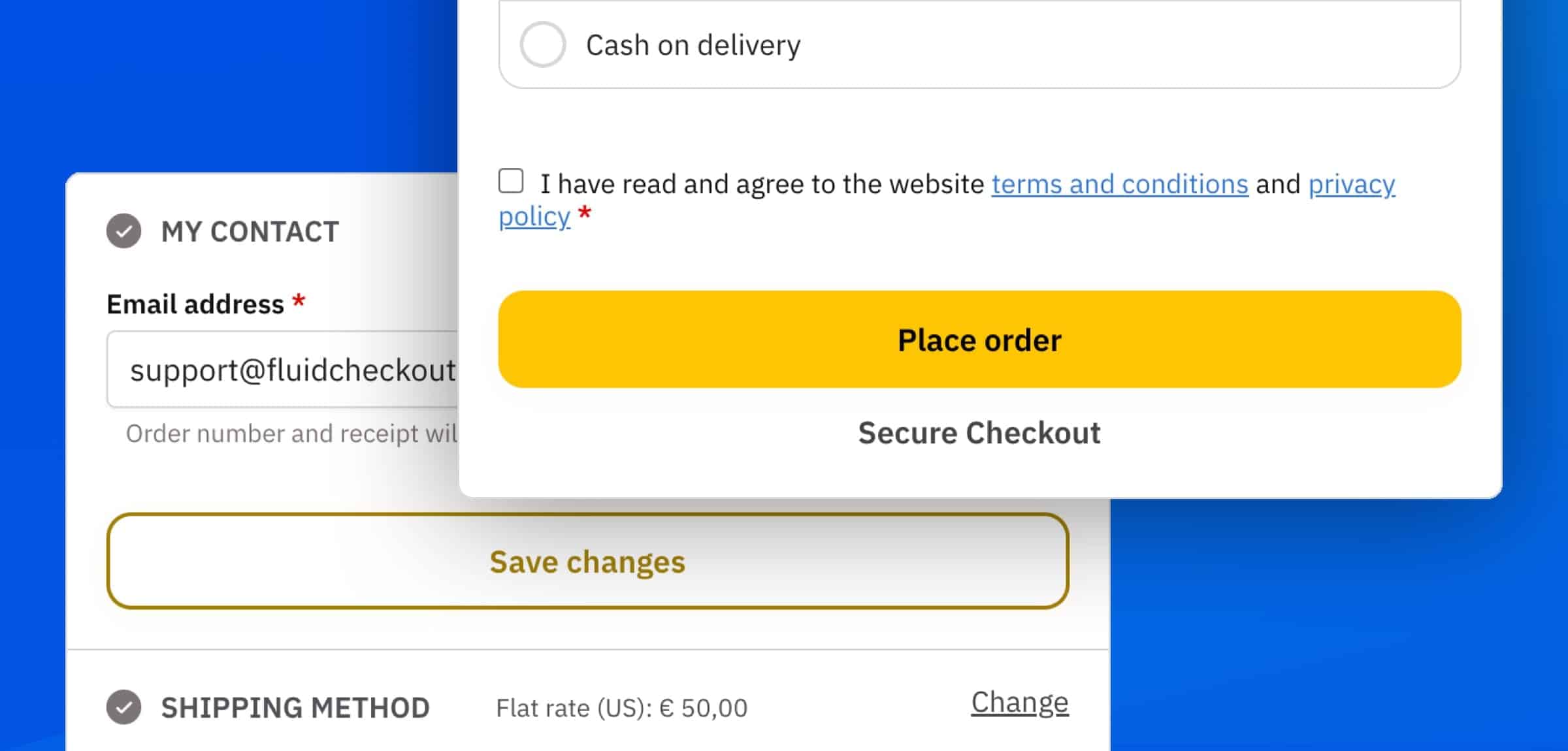
Since the introduction of design templates with Fluid Checkout Lite 3.0, it is possible to use CSS variables to easily change the colors of buttons on the checkout page, and on other pages optimized by Fluid Checkout PRO as the cart page, order received and view order pages.
Button colors for primary and secondary actions #
Fluid Checkout makes a distinction between buttons associated with primary actions and secondary actions on a page or section.
When customizing the button colors, it is possible to change the styles for these button types separately.
Primary actions are the most important action the user should take on that page or section of the page.
Secondary actions are all other actions the user can take on that same page or section.
Primary buttons | Secondary buttons |
---|---|
– Proceed to next step – Place order – Proceed to checkout, on the cart page | – Add to cart, on the cart page cross sells suggested products – Save changes, when editing one specific sub-step on the checkout page |
Please note that while saving the changes made to a sub-step on the checkout page is an importan action to take, the main action in the broader context would be to complete the purchase with the buttons “Place order”, or “Proceed to next step”.
Enable custom button styles via PHP #
To preserve as much of the theme styles as possible, the custom button styles will be disabled by default. You need to use a code snippet to start applying the button color and other styles changes to your checkout and other pages.
Use the code snippet below to enable applying the button color styles:
// Enable button color changes
add_filter( 'fc_apply_button_colors_styles', '__return_true', 10 );
Basically this code snippet will enable adding the class has-fc-button-colors
to the body element on the optimized pages, activating the necessary CSS code used to change the button colors.
If you need to change other button styles such as border, radius or shadows, you can use the code snippet below to enable applying other button design styles:
// Enable other button design changes
add_filter( 'fc_apply_button_design_styles', '__return_true', 10 );
If you are unsure about how to add the code snippet to your website, check our article:
How to safely add code snippets to your WooCommerce website.
Change button styles via PHP (recommended method) #
To change the button colors and other button styles, you can use the hook fc_css_variables
to set values for CSS variables used on all the pages optimized by Fluid Checkout Lite and Fluid Checkout PRO.
It is also possible to set multiple contexts with different values. Multiple contexts can be specially useful if your website allows users to choose between light and dark mode for example, yet they have other applications too.
Alternatively, you can also change CSS variables directly in your custom CSS code.
In any case, you need to enable the button styles using the hooks as described above in the section Enable custom button styles via PHP.
/**
* Change button colors.
*
* @param array $css_variables The CSS variables contexts with key/value pairs for each CSS variable.
* The contexts are defined with the CSS selectors to which the CSS variables should apply.
*/
function fluidcheckout_change_button_styles( $css_variables ) {
// Bail if design template class is not available
if ( ! class_exists( 'FluidCheckout_DesignTemplates' ) ) { return $css_variables; }
// Add CSS variables
$new_css_variables = array(
// The `:root` context applies to the entire page
//
// If you custom colors are not applying, you might need to change the context to be more specific.
// For example: `:root body.theme-shoptimizer` will override any CSS variables set to the `:root` context.
//
// Any valid CSS property value can be used for each CSS variable,
// provided they are also valid for the CSS property these variable are actually used on.
':root' => array(
// Primary button colors
'--fluidcheckout--button--primary--border-color' => '#fdc500',
'--fluidcheckout--button--primary--background-color' => '#fdc500',
'--fluidcheckout--button--primary--text-color' => '#000',
'--fluidcheckout--button--primary--border-color--hover' => '#ffd500',
'--fluidcheckout--button--primary--background-color--hover' => '#ffd500',
'--fluidcheckout--button--primary--text-color--hover' => '#000',
// Secondary button colors
'--fluidcheckout--button--secondary--border-color' => '#a38000',
'--fluidcheckout--button--secondary--background-color' => 'transparent',
'--fluidcheckout--button--secondary--text-color' => '#a38000',
'--fluidcheckout--button--secondary--border-color--hover' => '#222',
'--fluidcheckout--button--secondary--background-color--hover' => 'transparent',
'--fluidcheckout--button--secondary--text-color--hover' => '#222',
// Button design styles
'--fluidcheckout--button--height' => '50px',
'--fluidcheckout--button--border-width' => '2px',
'--fluidcheckout--button--border-style' => 'solid',
'--fluidcheckout--button--border-radius' => '13px',
'--fluidcheckout--button--box-shadow-color' => 'rgba( 253, 197, 0, .05 )',
'--fluidcheckout--button--box-shadow-blur-radius' => '20px',
'--fluidcheckout--button--font-size' => '16px',
'--fluidcheckout--button--font-weight' => 'bold',
),
);
return FluidCheckout_DesignTemplates::instance()->merge_css_variables( $css_variables, $new_css_variables );
}
add_action( 'fc_css_variables', 'fluidcheckout_change_button_styles', 100 );
// Enable button color changes
add_filter( 'fc_apply_button_colors_styles', '__return_true', 10 );
// Enable other button design changes
add_filter( 'fc_apply_button_design_styles', '__return_true', 10 );
If you are unsure about how to add the code snippet to your website, check our article:
How to safely add code snippets to your WooCommerce website.
When using this code snippet, the buttons on your checkout page will look like the first image on this page.
Change button styles via CSS variables directly #
Alternatively, you can change the styles of buttons using custom CSS code directly. When changing the CSS variables directly, you need to ensure your custom CSS code is added to all pages optimized by Fluid Checkout. Instead, when using the preferred method via the PHP hook fc_css_variables
that is already managed by Fluid Checkout.
In any case, you need to enable the button styles using the hooks as described above in the section Enable custom button styles via PHP.
/**
* The `:root` context applies to the entire page
*
* If you custom colors are not applying, you might need to change the context to be more specific.
* For example: `:root body.theme-shoptimizer` will override any CSS variables set to the `:root` context.
*
* Any valid CSS property value can be used for each CSS variable,
* provided they are also valid for the CSS property these variable are actually used on.
*/
:root {
/* Primary button colors */
--fluidcheckout--button--primary--border-color: #fdc500;
--fluidcheckout--button--primary--background-color: #fdc500;
--fluidcheckout--button--primary--text-color: #000;
--fluidcheckout--button--primary--border-color--hover: #ffd500;
--fluidcheckout--button--primary--background-color--hover: #ffd500;
--fluidcheckout--button--primary--text-color--hover: #000;
/* Secondary button colors */
--fluidcheckout--button--secondary--border-color: #a38000;
--fluidcheckout--button--secondary--background-color: transparent;
--fluidcheckout--button--secondary--text-color: #a38000;
--fluidcheckout--button--secondary--border-color--hover: #222;
--fluidcheckout--button--secondary--background-color--hover: transparent;
--fluidcheckout--button--secondary--text-color--hover: #222;
/* Button design styles */
--fluidcheckout--button--height: 50px;
--fluidcheckout--button--border-width: 2px;
--fluidcheckout--button--border-style: solid;
--fluidcheckout--button--border-radius: 13px;
--fluidcheckout--button--box-shadow-color: rgba( 253, 197, 0, .05 );
--fluidcheckout--button--box-shadow-blur-radius: 20px;
--fluidcheckout--button--font-size: 16px;
--fluidcheckout--button--font-weight: bold;
}
Please note that enabling the button color and design styles using the code snippet as mentioned above is still required for applying the button styles changes. Otherwise, you can also activate these styles by somehow adding the classes has-fc-button-colors
and has-fc-button-styles
to the body
element of the page.
// Enable button color changes
add_filter( 'fc_apply_button_colors_styles', '__return_true', 10 );
// Enable other button design changes
add_filter( 'fc_apply_button_design_styles', '__return_true', 10 );
If you are unsure about how to add the code snippet to your website, check our article:
How to safely add code snippets to your WooCommerce website.
When using this custom CSS code and this code snippet, the buttons on your checkout page will look like the first image on this page.