The case for mandatory house number on checkout forms #
When using Google Address Autocomplete to autofill the address fields, some customers forget to check or add a house number to their addresses. This problem causes issues with delivering the customer’s purchase as carriers might not be able to locate the correct address on the street.
The first thought would be to validate the “Street address” field and make sure that part of it can be recognized as the house number.
Well, this could work for the majority of addresses but not all of them. It turns out that house numbers are not always straightforward.
Depending on the country, the house number might come first or last when writing an address. Other times, the house number might be accompanied by non-digits characters such as letters and separators like /
. Also, many addresses do not have a house number at all, and it would be impossible for those customers to enter their correct addresses.
For those reasons and more, plugins like Google Address Autocomplete cannot force users to enter a house number in the “Street address” field by default.
One way to solve the problem is to create a new “House number” field and make it mandatory, and then map the “house number”.
In this case, if a house number value is not returned from the address suggestions or otherwise informed by the customer, that field will be empty, and the customer will not be able to move forward until a value is entered. For addresses that do not have a house number, the customer can be instructed to enter a value such as 0
or N/A
to indicate that.
Creating a new house number field and making it mandatory #
We’ll be using the plugin Checkout Field Editor for WooCommerce (by ThemeHigh) to create the new house number field, however, it should also be possible to use other similar plugins or custom code to make these changes.
If you are using the Google Address Autocomplete plugin, you’ll also need to map the value from the Google Places API to the newly created field.
1. Install the plugin Checkout Field Editor for WooCommerce (by ThemeHigh) and go to WP Admin > WooCommerce > Checkout Form.
2. Locate the “House number” field in the fields list, or click the button to “+ Add field” to create a new field in the Billing Fields group.
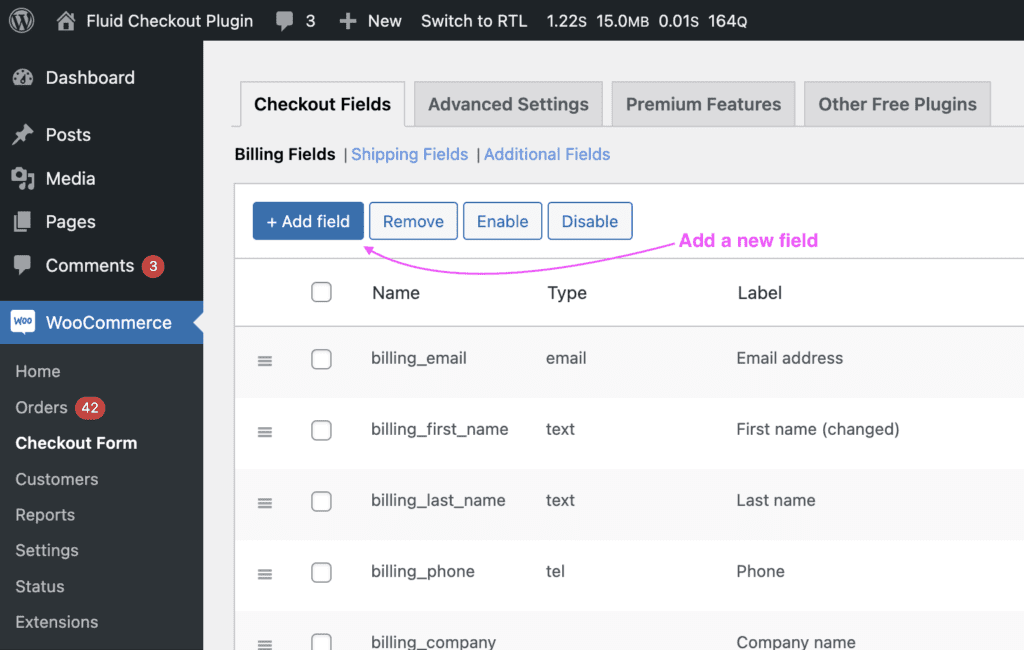
3. In the Edit field screen, select the field “Type” as “Text”. Remember to leave the “Validation” field empty, as house numbers might contain letter and other characters.
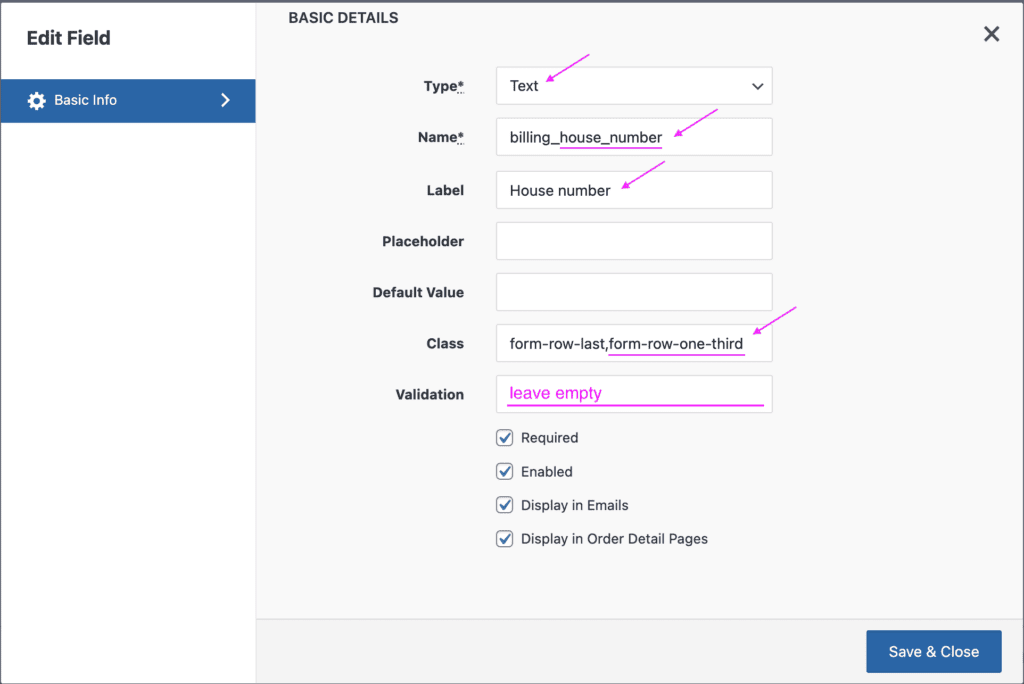
4. Give it an ID in the “Name” field that such as billing_house_number
. If you are using Fluid Checkout, please note that the field name endings should match on both the Billing and Shipping groups so that the option to set the Billing address to same as shipping address work as expected.
5. Set the field label that will be visible on the checkout page in the field “Label”, for instance “House number”.
6. Set the field classes to form-row-last
. If you are using Fluid Checkout on your website, you can also add the class form-row-one-third
to this field so it does not take too much space in the checkout form. These classes need to be separated with a comma ,
.
7. Set the field as “Required” if it is not yet set.
8. Click the “Save & Close” button to save the field.
9. Back to fields list in the Billing group, click to edit the billing_address_1
field.
10. In the Edit field screen, change the field “Label” to “Street name”.
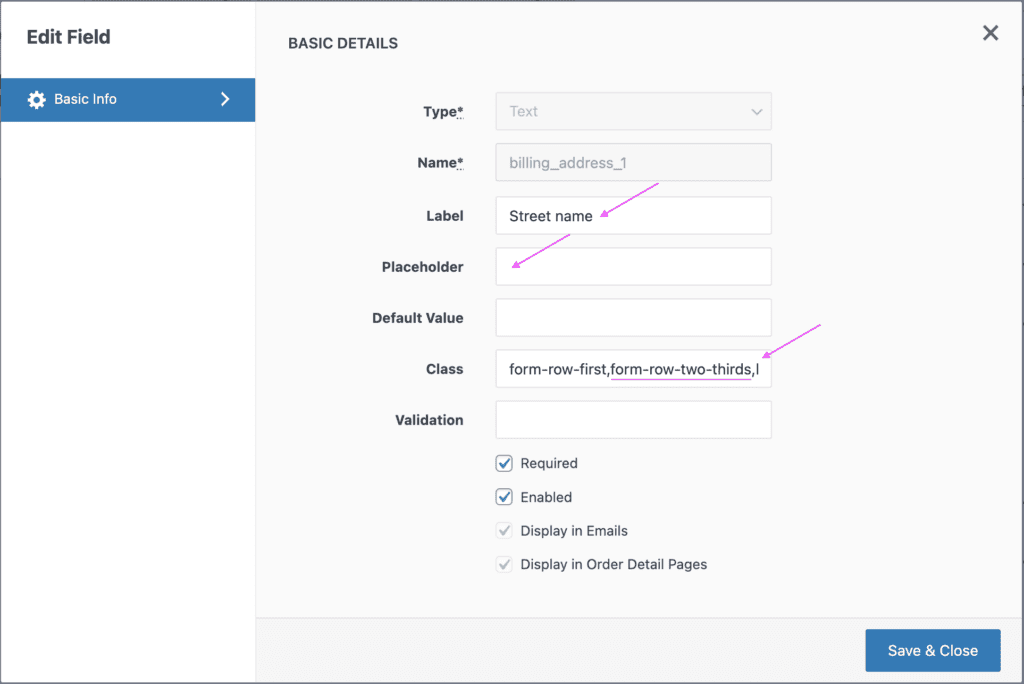
11. Remove the field “Placeholder” text if it is already set.
12. Set the field classes to form-row-first
. If you are using Fluid Checkout on your website, you can also add the class form-row-two-thirds
so that the “Street name” field takes two-thirds of the available space. These classes need to be separated with a comma ,
.
13. Click the “Save & Close” button to save the field.
14. Back to list of fields in the Billing group, move the new billing_house_number
field in between the fields billing_address_1
and billing_address_2
.

15. At the bottom of the page, click the button “Save changes” to save the new position for the “House number” field.
16. Repeat the process for the shipping address, adding the “House number” field in the Shipping group.
At this point, the address fields in your checkout page should look similar to this:
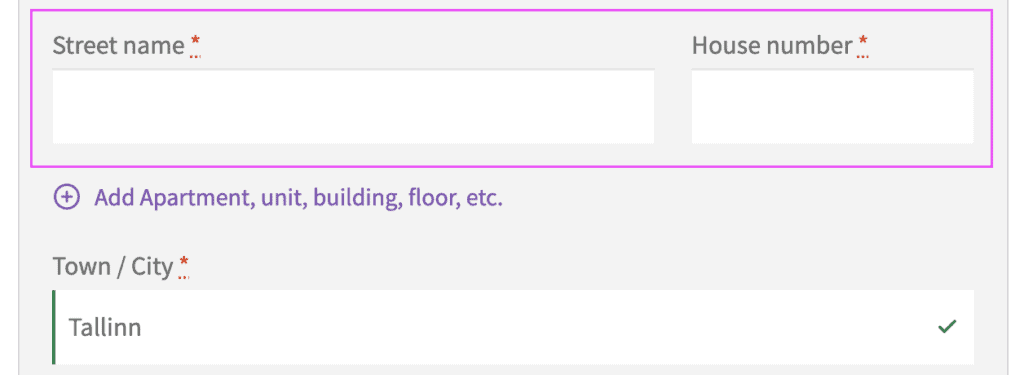
House number field validation #
Since house numbers might contain letter and other characters, depending on the country, remember to disable any validation on the custom house number field.
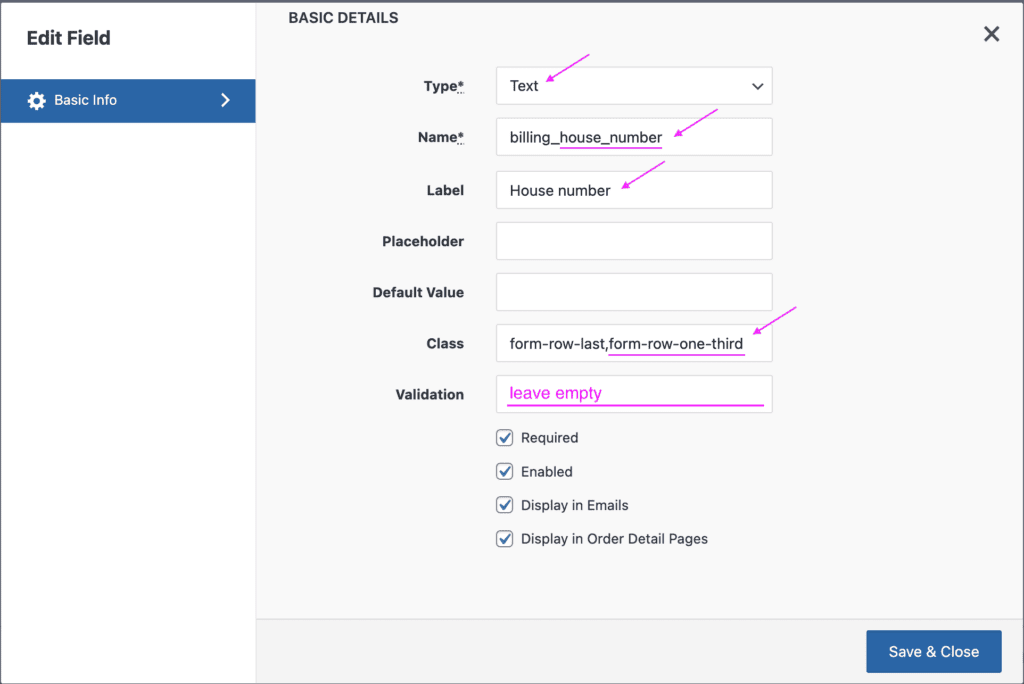
Compatibility with plugin Germanized #
In case you are also using the plugin Germanized, which validates the “Street address” (address_1
) field looking for a valid house number within it.
Remember to set the option “Validate street number” to “Never”. You can find this option at WP Admin > WooCommerce > Germanized > General > Checkout.
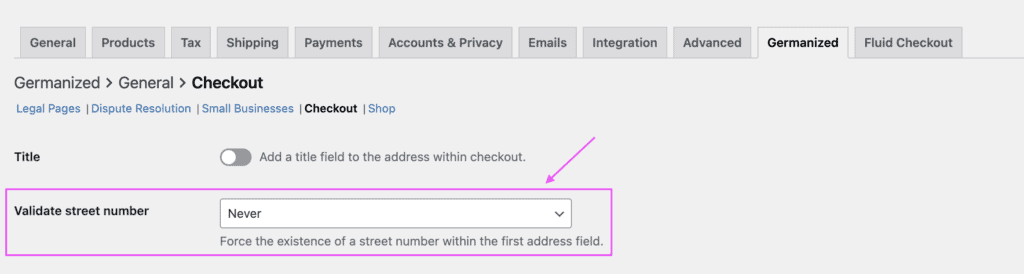
Mapping custom fields to the formatted address patterns #
For the house number to show correctly on the formatted address when then shipping and billing address steps are completed and on the order details pages, we need to tell WooCommerce what is the format for displaying addresses to include the custom fields, and how to get the value replacements for these custom fields.
Changing formatted addresses display format #
By default, WooCommerce will not know about the custom house number field and how it is supposed to be used when displaying the formatted addresses.
With the code snippet below, we can change the formatted address formats to add the custom house number field value and display it before or after the address_1
field or another designated relative field.
/**
* Change formatted address formats to include the house number field.
*
* @param array $formats Default localisation formats.
*/
function fluidcheckout_change_address_formats( $formats ) {
// Define position mapping for house number field
$relative_field_mapping = array( 'default' => 'address_1' );
// Define position mapping for house number field
$components_separator_per_locale = array(
'default' => ' ',
'AD' => ', ',
'BR' => ', ',
'ES' => ', ',
'RU' => ', ',
'SM' => ', ',
);
// Locales for which the house number is displayed after the relative field (usually `address_1`).
// May not include all countries for which the house number is displayed after the relative field,
// in which case you will need to add that country to this list.
$locales_add_after = array( 'AE', 'AM', 'AU', 'AZ', 'BH', 'BI', 'BL', 'BN', 'BR', 'BW', 'BZ', 'CA', 'CG', 'CM', 'CN', 'CU', 'EG', 'FJ', 'FM', 'FR', 'GE', 'GF', 'GP', 'GU', 'GY', 'HN', 'IN', 'IR', 'IT', 'JM', 'KE', 'KG', 'KH', 'KW', 'KY', 'LK', 'LU', 'MC', 'MA', 'ME', 'MG', 'ML', 'MQ', 'MT', 'MU', 'MV', 'MY', 'NC', 'NG', 'NI', 'NZ', 'PG', 'PH', 'PR', 'RE', 'SA', 'SG', 'SL', 'SN', 'SX', 'SY', 'TH', 'TN', 'TT', 'GB', 'US', 'VE', 'VI', 'VN', 'ZA', 'ZW' );
// Define format replacement part for the house number field
$house_number_replacement = '{house_number}';
// Iterate over registered formats
foreach ( $formats as $locale => $format ) {
// Get position mapping
$position = in_array( $locale, $locales_add_after ) ? 'after' : 'before';
$relative_field = array_key_exists( $locale, $relative_field_mapping ) ? $relative_field_mapping[ $locale ] : $relative_field_mapping[ 'default' ];
// Get components separator for the locale
$components_separator = array_key_exists( $locale, $components_separator_per_locale ) ? $components_separator_per_locale[ $locale ] : $components_separator_per_locale[ 'default' ];
// Define format string replacement values
$replace = '{' . $relative_field . '}';
$with = 'before' === $position ? $house_number_replacement . $components_separator . $replace : $replace . $components_separator . $house_number_replacement;
// Insert the house number replacement into the locale address format
$format = str_replace( $replace, $with, $format );
$formats[ $locale ] = $format;
}
return $formats;
}
add_filter( 'woocommerce_localisation_address_formats', 'fluidcheckout_change_address_formats', 300 );
Please note that the code snippet above may not include all countries for which the house number is displayed after the relative field (usually address_1
), in which case you will need to add that country code to the list $locales_add_after
for this to work as expected for that country. If you find out that a country is missing on this list, please let us know and we’ll update the code snippet above with it.
Mapping custom field replacement values #
Since Fluid Checkout Lite 3.1.6+, you can use filter hook fc_formatted_address_replacements_custom_field_keys
to tell WooCommerce to replace the markers of custom fields on the address formats with the current values of those fields.
For the custom house number field, you can use the code snippet below.
If you are unsure about how to add the code snippet, check our article:
How to safely add code snippets to your WooCommerce website
/**
* Add custom fields for the formatted addresses replacements.
*
* @param array $replacements Custom field keys to be added to formatted address replacements.
*/
function fluidcheckout_add_custom_fields_for_formatted_address_replacements( $custom_field_keys ) {
// Add custom fields
$custom_field_keys = array_merge( $custom_field_keys, array(
'house_number',
) );
return $custom_field_keys;
}
add_filter( 'fc_formatted_address_replacements_custom_field_keys', 'fluidcheckout_add_custom_fields_for_formatted_address_replacements', 300 );
This will also tell Fluid Checkout to ignore these custom fields when adding them below the formatted address on the completed steps.
Mapping address suggestion results from the Google Address Autocomplete add-on to the custom house number field #
The checkout form should now have the new “House number” field added, however, the Google Address Autocomplete add-on does not know about it yet.
You need to use a code snippet to tell the plugin Google Address Autocomplete about the new field — or any custom field for that matter.
If you are unsure about how to add the code snippet, check our article:
How to safely add code snippets to your WooCommerce website
The code snippet below will change the default
field mappings, telling the plugin to autofill the new “House number” field with the value returned from the Google Places API. It also includes the same change specific to one country (US) that is commented out, it can be changed to any other country in case it does not work as some countries have different settings.
/**
* Change the `localeComponents` settings to fill custom house number fields.
*
* @param array $settings JS settings object of the plugin.
*/
function fcgaa_change_locale_mapping_house_number( $settings ) {
// New locale components to merge
$new_locale_components = array(
'default' => array(
'address_1' => array( 'route' ),
'house_number' => array( 'street_number' ),
'components_separator' => ', ',
),
// Example to set changes to specific countries only
// 'US' => array(
// 'address_1' => array( 'route' ),
// 'house_number' => array( 'street_number' ),
// 'components_separator' => ', ',
// ),
);
foreach ( $new_locale_components as $locale_key => $locale_settings ) {
// Create locale settings if not existent
if ( ! array_key_exists( $locale_key, $settings[ 'localeComponents' ] ) ) { $settings[ 'localeComponents' ][ $locale_key ] = array(); }
// Merge settings
$settings[ 'localeComponents' ][ $locale_key ] = array_merge( $settings[ 'localeComponents' ][ $locale_key ], $locale_settings );
}
return $settings;
}
add_filter( 'fc_gaa_google_autocomplete_js_settings', 'fcgaa_change_locale_mapping_house_number', 10 );
If you used a different field ID for the house number fields, you’ll need to adjust it in the field mapping code above. Please note that field IDs in the address suggestion data mapping are used without the group prefixes `billing_`
or `shipping_`
.