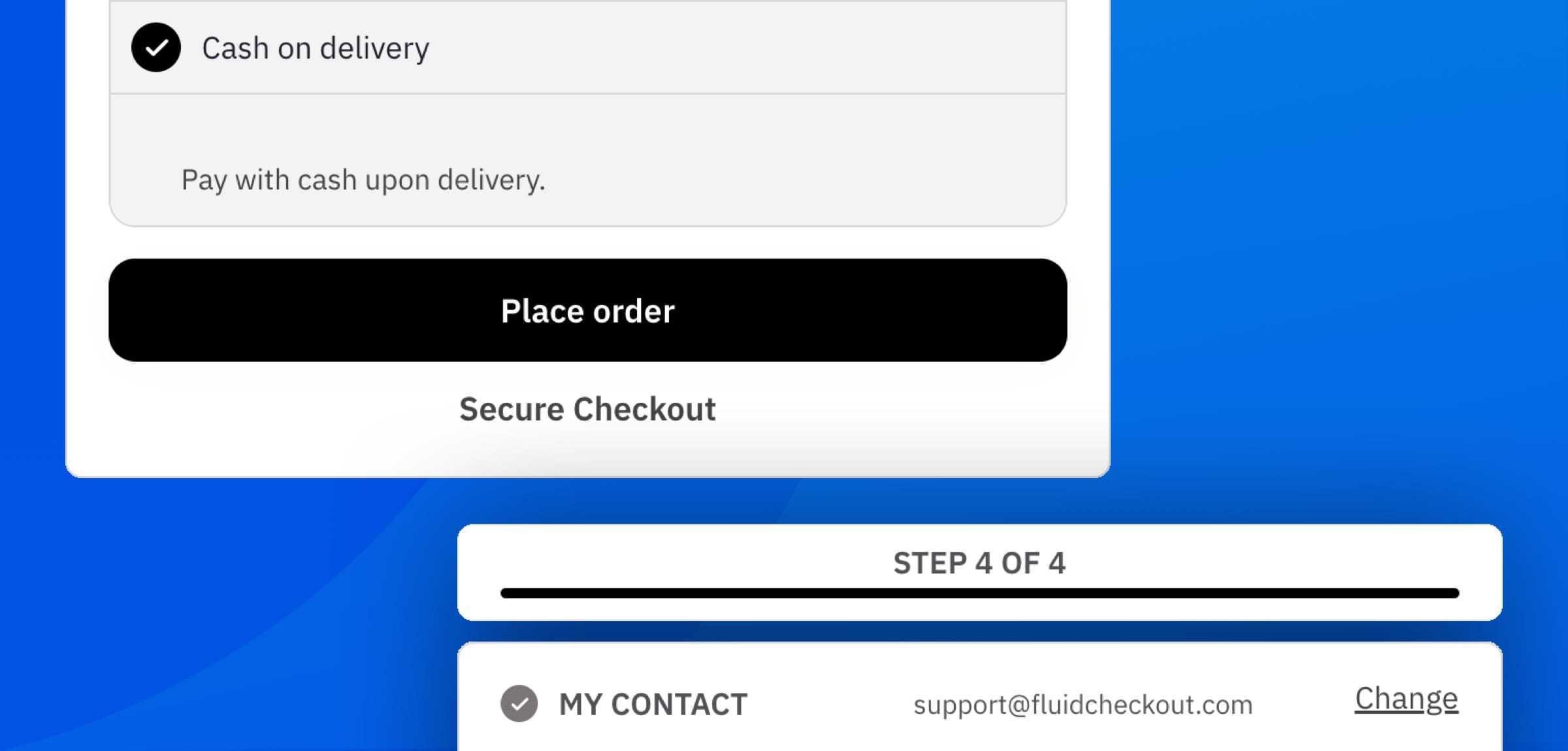
Since the introduction of design templates with Fluid Checkout Lite 3.0, it is possible to use CSS variables to easily change the colors of many elements on the checkout page, and on other pages optimized by Fluid Checkout PRO as the cart page, order received and view order pages.
Change colors via PHP hooks (recommended method) #
To change colors of Fluid Checkout elements, you can use the hook fc_css_variables
to set values for CSS variables used on all the pages optimized by Fluid Checkout Lite and Fluid Checkout PRO.
It is also possible to set multiple contexts with different values. Multiple contexts can be specially useful if your website allows users to choose between light and dark mode for example, yet they have other applications too.
In this article, you can find specific examples for changing the colors for each elements. Use the links below to jump to the elements you want to change the colors for:
- Base colors
- Header colors
- Progress bar colors
- Steps and other sections colors
- Order summary colors
- Button colors and other styles
- Shipping methods, payment methods and other option boxes colors
- Coupon codes colors
Alternatively, you can also change CSS variables directly in your custom CSS code.
Base colors #
Base colors are used on many elements and througout all pages optimized by Fluid Checkout. They are separated into a few types:
- Greys: Scale of greys from
black
towhite
with 5 variations in between. These colors are used everywhere, from backgrounds to text, border as and other elements. - Utility: Utility colors are used for implying an element is related to “success”, “error”, “alert” or “information”.
- Borders: Define the color for borders applied to many elements. Changing these colors will affect all elements where these colors are used, however, it is possible to change the color of borders for specific elements too.
- Shadows: Colors used for shadows for some elements depending on the design template used.
/**
* Change CSS variables for base colors.
*
* @param array $css_variables The CSS variables contexts with key/value pairs for each CSS variable.
* The contexts are defined with the CSS selectors to which the CSS variables should apply.
*/
function fluidcheckout_change_base_color_css_variables( $css_variables ) {
// Bail if design template class is not available
if ( ! class_exists( 'FluidCheckout_DesignTemplates' ) ) { return $css_variables; }
// Add CSS variables
$new_css_variables = array(
// The `:root` context applies to the entire page
//
// If you custom colors are not applying, you might need to change the context to be more specific.
// For example: `:root body.theme-shoptimizer` will override any CSS variables set to the `:root` context.
//
// Any valid CSS property value can be used for each CSS variable,
// provided they are also valid for the CSS property these variable are actually used on.
':root' => array(
// Base greys
'--fluidcheckout--color--black' => '#000',
'--fluidcheckout--color--darker-grey' => '#1E212B',
'--fluidcheckout--color--dark-grey' => '#535156',
'--fluidcheckout--color--grey' => '#7b7575',
'--fluidcheckout--color--light-grey' => '#d8d8d8',
'--fluidcheckout--color--lighter-grey' => '#f3f3f3',
'--fluidcheckout--color--white' => '#fff',
// Base utility colors
'--fluidcheckout--color--success' => '#007a3d',
'--fluidcheckout--color--error' => '#cc1818',
'--fluidcheckout--color--alert' => '#c95000',
'--fluidcheckout--color--info' => '#1f01b9',
// Base border colors
'--fluidcheckout--border-color' => '#d8d8d8',
'--fluidcheckout--border-color--dark' => '#7b7575',
'--fluidcheckout--border-color--light' => '#f3f3f3',
// Base shadow colors
'--fluidcheckout--shadow-color--darker' => 'rgba( 0, 0, 0, .30 )',
'--fluidcheckout--shadow-color--dark' => 'rgba( 0, 0, 0, .15 )',
),
);
return FluidCheckout_DesignTemplates::instance()->merge_css_variables( $css_variables, $new_css_variables );
}
add_action( 'fc_css_variables', 'fluidcheckout_change_base_color_css_variables', 100 );
If you are unsure about how to add the code snippet to your website, check our article:
How to safely add code snippets to your WooCommerce website.
Header colors #
Use the code snippet below to change the colors for the distraction free page header for the checkout and cart pages.
/**
* Change CSS variables for headers.
*
* @param array $css_variables The CSS variables contexts with key/value pairs for each CSS variable.
* The contexts are defined with the CSS selectors to which the CSS variables should apply.
*/
function fluidcheckout_change_header_color_css_variables( $css_variables ) {
// Bail if design template class is not available
if ( ! class_exists( 'FluidCheckout_DesignTemplates' ) ) { return $css_variables; }
// Add CSS variables
$new_css_variables = array(
// The `:root` context applies to the entire page
//
// If you custom colors are not applying, you might need to change the context to be more specific.
// For example: `:root body.theme-shoptimizer` will override any CSS variables set to the `:root` context.
//
// Any valid CSS property value can be used for each CSS variable,
// provided they are also valid for the CSS property these variable are actually used on.
':root' => array(
// Header colors
'--fluidcheckout--header--background-color' => '#fff',
'--fluidcheckout--header--shadow-color' => 'rgba( 0, 0, 0, .15 )',
'--fluidcheckout--header--shadow-color--large-screen' => 'transparent',
),
);
return FluidCheckout_DesignTemplates::instance()->merge_css_variables( $css_variables, $new_css_variables );
}
add_action( 'fc_css_variables', 'fluidcheckout_change_header_color_css_variables', 100 );
If you are unsure about how to add the code snippet to your website, check our article:
How to safely add code snippets to your WooCommerce website.
Progress bar colors #
Use the code snippet below to change the colors for the progress bar on the checkout page.
/**
* Change CSS variables for the progress bar.
*
* @param array $css_variables The CSS variables contexts with key/value pairs for each CSS variable.
* The contexts are defined with the CSS selectors to which the CSS variables should apply.
*/
function fluidcheckout_change_progress_bar_color_css_variables( $css_variables ) {
// Bail if design template class is not available
if ( ! class_exists( 'FluidCheckout_DesignTemplates' ) ) { return $css_variables; }
// Add CSS variables
$new_css_variables = array(
// The `:root` context applies to the entire page
//
// If you custom colors are not applying, you might need to change the context to be more specific.
// For example: `:root body.theme-shoptimizer` will override any CSS variables set to the `:root` context.
//
// Any valid CSS property value can be used for each CSS variable,
// provided they are also valid for the CSS property these variable are actually used on.
':root' => array(
// Progress bar colors
'--fluidcheckout--checkout-progress--background-color' => '#fff',
'--fluidcheckout--checkout-progress--bar-color' => '#d8d8d8',
'--fluidcheckout--checkout-progress--bar-color--complete' => '#007a3d',
'--fluidcheckout--checkout-progress--bar-color--current' => '#007a3d',
'--fluidcheckout--checkout-progress--step-count--text-color' => '#535156',
),
);
return FluidCheckout_DesignTemplates::instance()->merge_css_variables( $css_variables, $new_css_variables );
}
add_action( 'fc_css_variables', 'fluidcheckout_change_progress_bar_color_css_variables', 100 );
If you are unsure about how to add the code snippet to your website, check our article:
How to safely add code snippets to your WooCommerce website.
Steps, cart sections and order details sections colors #
Use the code snippet below to change the colors for the checkout steps, cart sections, and sections on the order details pages.
/**
* Change CSS variables for sections.
*
* @param array $css_variables The CSS variables contexts with key/value pairs for each CSS variable.
* The contexts are defined with the CSS selectors to which the CSS variables should apply.
*/
function fluidcheckout_change_section_color_css_variables( $css_variables ) {
// Bail if design template class is not available
if ( ! class_exists( 'FluidCheckout_DesignTemplates' ) ) { return $css_variables; }
// Add CSS variables
$new_css_variables = array(
// The `:root` context applies to the entire page
//
// If you custom colors are not applying, you might need to change the context to be more specific.
// For example: `:root body.theme-shoptimizer` will override any CSS variables set to the `:root` context.
//
// Any valid CSS property value can be used for each CSS variable,
// provided they are also valid for the CSS property these variable are actually used on.
':root' => array(
// Section colors
'--fluidcheckout--section--background-color' => '#fff',
'--fluidcheckout--section--border-color' => '#d8d8d8',
'--fluidcheckout--section--highlighted-background-color' => '#f3f3f3',
'--fluidcheckout--section--highlighted-field-background-color' => '#fff',
),
);
return FluidCheckout_DesignTemplates::instance()->merge_css_variables( $css_variables, $new_css_variables );
}
add_action( 'fc_css_variables', 'fluidcheckout_change_section_color_css_variables', 100 );
If you are unsure about how to add the code snippet to your website, check our article:
How to safely add code snippets to your WooCommerce website.
Order summary colors #
Use the code snippet below to change the colors for the order summary on the checkout, cart and order details pages.
/**
* Change CSS variables for the order summary.
*
* @param array $css_variables The CSS variables contexts with key/value pairs for each CSS variable.
* The contexts are defined with the CSS selectors to which the CSS variables should apply.
*/
function fluidcheckout_change_order_summary_color_css_variables( $css_variables ) {
// Bail if design template class is not available
if ( ! class_exists( 'FluidCheckout_DesignTemplates' ) ) { return $css_variables; }
// Add CSS variables
$new_css_variables = array(
// The `:root` context applies to the entire page
//
// If you custom colors are not applying, you might need to change the context to be more specific.
// For example: `:root body.theme-shoptimizer` will override any CSS variables set to the `:root` context.
//
// Any valid CSS property value can be used for each CSS variable,
// provided they are also valid for the CSS property these variable are actually used on.
':root' => array(
// Progress bar colors
'--fluidcheckout--checkout-progress--background-color' => '#fff',
'--fluidcheckout--checkout-progress--bar-color' => '#d8d8d8',
'--fluidcheckout--checkout-progress--bar-color--complete' => '#007a3d',
'--fluidcheckout--checkout-progress--bar-color--current' => '#007a3d',
'--fluidcheckout--checkout-progress--step-count--text-color' => '#535156',
// Order summary shadow colors
'--fluidcheckout--order-summary--shadow-color' => 'transparent',
'--fluidcheckout--order-summary--shadow-color--large-screen' => 'transparent',
// Order summary section colors
'--fluidcheckout--order-summary--border-color' => '#d8d8d8',
'--fluidcheckout--order-summary--background-color' => '#fff',
'--fluidcheckout--order-summary--background-color--popup' => '#fff',
// Order summary table cell colors
'--fluidcheckout--order-summary--cell-border-color' => '#d8d8d8',
'--fluidcheckout--order-summary--cell-border-color--products-list' => '#d8d8d8',
'--fluidcheckout--order-summary--cell-border-color--products' => '#d8d8d8',
'--fluidcheckout--order-summary--cell-border-color--summary' => '#d8d8d8',
'--fluidcheckout--order-summary--cell-border-color--totals-top' => '#d8d8d8',
'--fluidcheckout--order-summary--cell-border-color--totals-bottom' => '#d8d8d8',
'--fluidcheckout--order-summary--highlighted-total-background-color' => '#f3f3f3',
// Order summary product image colors
'--fluidcheckout--order-summary--image-background-color' => '#fff',
'--fluidcheckout--order-summary--image-border-color' => '#d8d8d8',
'--fluidcheckout--order-summary--image-shadow-color' => 'transparent',
// Order summary quantity text colors
'--fluidcheckout--order-summary--quantity-background-color' => '#535156',
'--fluidcheckout--order-summary--quantity-text-color' => 'fff',
),
);
return FluidCheckout_DesignTemplates::instance()->merge_css_variables( $css_variables, $new_css_variables );
}
add_action( 'fc_css_variables', 'fluidcheckout_change_order_summary_color_css_variables', 100 );
If you are unsure about how to add the code snippet to your website, check our article:
How to safely add code snippets to your WooCommerce website.
Button colors and other styles #
To preserve as much as possible of the button styles from the theme styles, the custom button styles will be disabled by default.
Since customizing buttons is a bit more complex than the colors of other elements, we have a separate guide for that.
Go to our our dedicated documentation on how to customize button colors and other button styles.
Shipping methods, payment methods and other option boxes colors #
Use the code snippet below to change the colors for the shipping methods, payment methods, and other similar option boxes.
/**
* Change CSS variables for option boxes.
*
* @param array $css_variables The CSS variables contexts with key/value pairs for each CSS variable.
* The contexts are defined with the CSS selectors to which the CSS variables should apply.
*/
function fluidcheckout_change_option_box_color_css_variables( $css_variables ) {
// Bail if design template class is not available
if ( ! class_exists( 'FluidCheckout_DesignTemplates' ) ) { return $css_variables; }
// Add CSS variables
$new_css_variables = array(
// The `:root` context applies to the entire page
//
// If you custom colors are not applying, you might need to change the context to be more specific.
// For example: `:root body.theme-shoptimizer` will override any CSS variables set to the `:root` context.
//
// Any valid CSS property value can be used for each CSS variable,
// provided they are also valid for the CSS property these variable are actually used on.
':root' => array(
// Option box border colors
'--fluidcheckout--option-box--border-color' => '#d8d8d8',
'--fluidcheckout--option-box--border-color--hover' => '#7b7575',
// Option box label colors
'--fluidcheckout--option-box--label-color--checked' => '#1E212B',
'--fluidcheckout--option-box--label-border-color' => '#d8d8d8',
'--fluidcheckout--option-box--label-border-color--hover' => '#7b7575',
'--fluidcheckout--option-box--label-border-color--checked' => '#d8d8d8',
// Option box background colors
'--fluidcheckout--option-box--background-color' => '#fff',
'--fluidcheckout--option-box--background-color--hover' => '#f3f3f3',
'--fluidcheckout--option-box--background-color--checked' => '#f3f3f3',
// Option box radio button colors
'--fluidcheckout--option-box--radio-color' => '#d8d8d8',
'--fluidcheckout--option-box--radio-color--hover' => '#d8d8d8',
'--fluidcheckout--option-box--radio-color--checked' => '#007a3d',
'--fluidcheckout--option-box--radio-icon-color--checked' => '#fff',
),
);
return FluidCheckout_DesignTemplates::instance()->merge_css_variables( $css_variables, $new_css_variables );
}
add_action( 'fc_css_variables', 'fluidcheckout_change_option_box_color_css_variables', 100 );
If you are unsure about how to add the code snippet to your website, check our article:
How to safely add code snippets to your WooCommerce website.
Coupon codes colors #
Use the code snippet below to change the colors for the coupon codes items added to the cart. Please note that these colors do not apply for the coupon code fields.
<?php
/**
* Change CSS variables for coupon codes.
*
* @param array $css_variables The CSS variables contexts with key/value pairs for each CSS variable.
* The contexts are defined with the CSS selectors to which the CSS variables should apply.
*/
function fluidcheckout_change_coupon_code_css_variables( $css_variables ) {
// Bail if design template class is not available
if ( ! class_exists( 'FluidCheckout_DesignTemplates' ) ) { return $css_variables; }
// Add CSS variables
$new_css_variables = array(
// The `:root` context applies to the entire page
//
// If you custom colors are not applying, you might need to change the context to be more specific.
// For example: `:root body.theme-shoptimizer` will override any CSS variables set to the `:root` context.
//
// Any valid CSS property value can be used for each CSS variable,
// provided they are also valid for the CSS property these variable are actually used on.
':root' => array(
// Coupon code items styles
'--fluidcheckout--coupon-code--background-color' => 'transparent',
'--fluidcheckout--coupon-code--border-color' => '#d8d8d8',
'--fluidcheckout--coupon-code--border-style' => 'solid',
'--fluidcheckout--coupon-code--border-radius' => '0',
// Coupon code section styles
'--fluidcheckout--discount-section--border-style' => 'solid',
'--fluidcheckout--discount-section--border-color' => 'gold',
),
);
return FluidCheckout_DesignTemplates::instance()->merge_css_variables( $css_variables, $new_css_variables );
}
add_action( 'fc_css_variables', 'fluidcheckout_change_coupon_code_css_variables', 100 );
If you are unsure about how to add the code snippet to your website, check our article:
How to safely add code snippets to your WooCommerce website.
Change colors via CSS variables directly #
Alternatively, you can change CSS variables using custom CSS code directly. When changing the CSS variables directly, you need to ensure your custom CSS code is added to all pages optimized by Fluid Checkout. Instead, when using the preferred method via the PHP hook fc_css_variables
that is already managed by Fluid Checkout.
/**
* The `:root` context applies to the entire page
*
* If you custom colors are not applying, you might need to change the context to be more specific.
* For example: `:root body.theme-shoptimizer` will override any CSS variables set to the `:root` context.
*
* Any valid CSS property value can be used for each CSS variable,
* provided they are also valid for the CSS property these variable are actually used on.
*/
:root {
/* Base greys */
--fluidcheckout--color--black: #000;
--fluidcheckout--color--darker-grey: #1E212B;
--fluidcheckout--color--dark-grey: #535156;
--fluidcheckout--color--grey: #7b7575;
--fluidcheckout--color--light-grey: #d8d8d8;
--fluidcheckout--color--lighter-grey: #f3f3f3;
--fluidcheckout--color--white: #fff;
// Add more CSS variables here.
}