With the Local Pickup feature, customers who choose a local pickup shipping method during checkout will not have to enter their shipping address. By default, this Fluid Checkout PRO feature supports only one local pickup shipping method which will be associated with the main shop address configured in the WooCommerce General settings.
In case customers should pick up their orders on a different address than your main shop address as configured in the WooCommerce General settings, or if your shop offers more than one pickup location, you can follow the instructions below to customize the pickup location address or configure multiple pickup locations if that is the case.
Customize pickup location address #
By default, the Local Pickup feature will use the main shop address as configured in the WooCommerce General settings.
Before proceeding with customizing the local pickup address with the code snippet below, check whether you can change your main shop address at WP Admin > WooCommerce > Settings > General.
In case you cannot change your main shop address, use the code snippet below to customize the local pickup location address that is be displayed on the checkout page, and on the order details after an order is placed by the customer.
/**
* Change local pickup point address data for single pickup location.
*
* @param array $address_data The address data for the pickup location.
*/
function fluidcheckout_change_pickup_point_address_data( $address_data ) {
$address_data = array(
'company' => 'Amsterdam Shop',
'address_1' => '1013 Hermitagelaan',
'address_2' => '2nd floor',
'city' => 'Amsterdam',
'state' => 'Noord-Holland',
'country' => 'NL',
'postcode' => '1064WE',
);
return $address_data;
}
add_filter( 'fc_pro_pickup_point_address_data', 'fluidcheckout_change_pickup_point_address_data', 10 );
If you are unsure about how to add the code snippet to your website, check our article:
How to safely add code snippets to your WooCommerce website
Use multiple pickup locations #
With the Local Pickup feature, it is possible to use multiple local pickup shipping methods to offer customers more options of locations where they can pickup their orders. After adding multiple local pickup shipping methods, you can customize the pickup location address associated with each shipping method.
Add multiple local pickup shipping method in the WooCommerce settings #
In WooCommerce, you can configure multiple local pickup shipping methods in the built-in settings. Here’s a step-by-step guide on how to add multiple local pickup shipping methods:
- Go to WP Admin > WooCommerce > Settings > Shipping > Shipping zones.
- Choose a shipping zone to add the local pickup shipping methods to and click its name. Please observe that currently there are some limitations to how you can configure shipping zones to work with the Local Pickup feature — see documentation.
- On the shipping zone settings, you can see and manage the shipping methods available for that shipping zone.
- Click the option “Add shipping method” to add more local pickup shipping methods if needed.
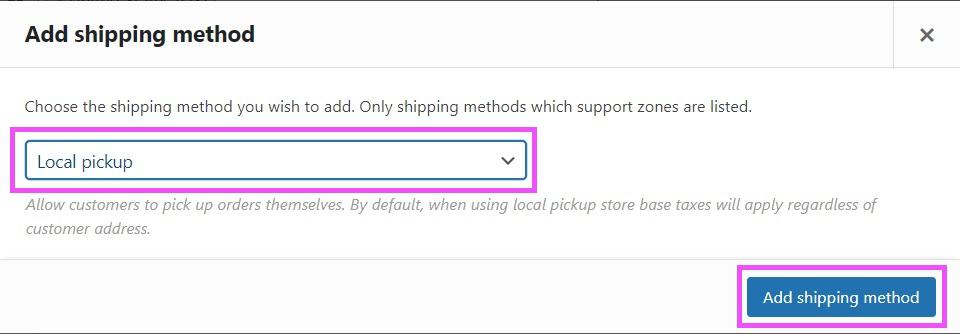
- Make sure to select the native shipping method type “Local pickup”, then click the option “Add shipping method” on the popup screen.
- Locate the shipping method that was just added and click on its name (probably “Local pickup” at this point) or the option “Edit” that appears when you hover the mouse over it.
- Give it a descriptive title to suggest where which pickup location it is (ie. “Amsterdam Shop”), and set its taxable status and cost if any.
- Save the changes to the shipping method.
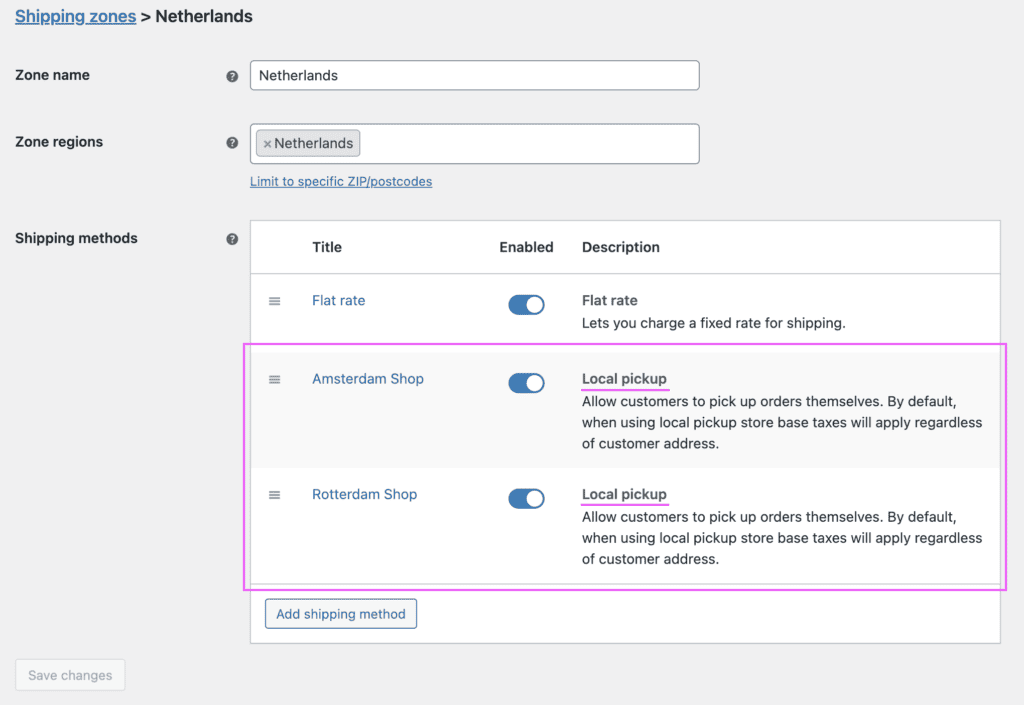
- Repeat these steps to add as many local pickup shipping methods as needed.
- Test on the checkout page that these shipping methods are displayed.
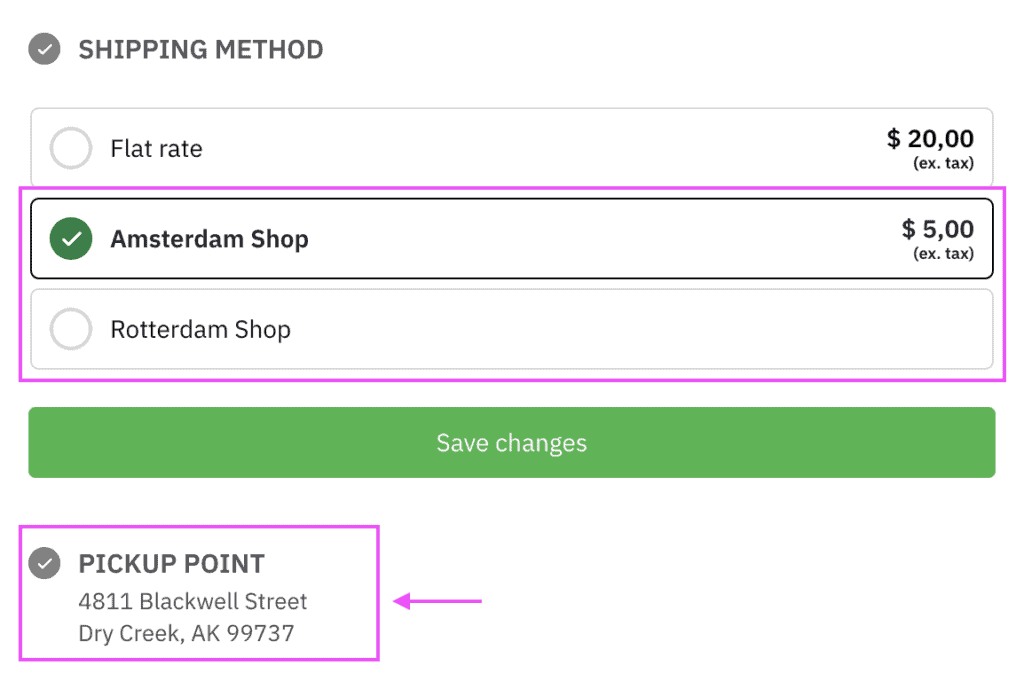
At this point, all these local pickup shipping methods will be using the main shop address as configured in the WooCommerce General settings as the pickup location. Follow the steps in the section Customize pickup location address based on selected shipping method below to dynamically change the pickup location address based on the shipping method selected at checkout.
In case the “Shipping to” section on the checkout page does not get hidden and replaced with the section “Pickup location”, make sure you have enabled the Local Pickup feature on the plugin settings.
Customize pickup location address based on selected shipping method #
When you offer your customers multiple pickup locations, you might need to dynamically change the pickup location based on the selected shipping method, otherwise the main shop location will be used for all local pickup options.
In order to properly configure the correct addresses for each pickup location you offer, you will need to customize the code snippet below with that information.
You will also need to find the shipping method ID for each pickup location to be able to associate the location address with the each local pickup shipping method you offer. Follow the instructions on the section Finding the local pickup shipping method id below to identify each shipping method ID.
/**
* Change local pickup point address data based on the shipping method selected.
*
* @param array $address_data The address data for the pickup location.
*/
function fluidcheckout_change_pickup_point_address_data_for_shipping_method( $address_data ) {
// Get seleted shipping method
$chosen_method = isset( WC()->session->chosen_shipping_methods[0] ) ? WC()->session->chosen_shipping_methods[0] : '';
// Define pickup addresses
$pickup_addresses = array(
// Default to an empty address
'' => array(),
// Location 1
// Change in the line below the shipping method ID to match that on your website
'local_pickup:14' => array(
// Change the pickup location address below
// adding or removing the line for any information as needed.
'company' => 'Amsterdam Shop',
'address_1' => '1013 Hermitagelaan',
'address_2' => '2nd floor',
'city' => 'Amsterdam',
'state' => 'Noord-Holland',
'country' => 'NL',
'postcode' => '1064WE',
),
// Location 2
// Change in the line below the shipping method ID to match that on your website
'local_pickup:17' => array(
// Change the pickup location address below
// adding or removing the line for any information as needed.
'company' => 'Roterdam Shop',
'address_1' => '66C, Sluisjesdijk',
'address_2' => '34th floor',
'city' => 'Roterdam',
'state' => 'South Holland',
'country' => 'NL',
'postcode' => '3087 AJ',
),
// [add more locations as needed]
);
// Get address data for the chosen shipping method
$address_data = $pickup_addresses[ $chosen_method ];
return $address_data;
}
add_filter( 'fc_pro_pickup_point_address_data', 'fluidcheckout_change_pickup_point_address_data_for_shipping_method', 10 );
If you are unsure about how to add the code snippet to your website, check our article:
How to safely add code snippets to your WooCommerce website
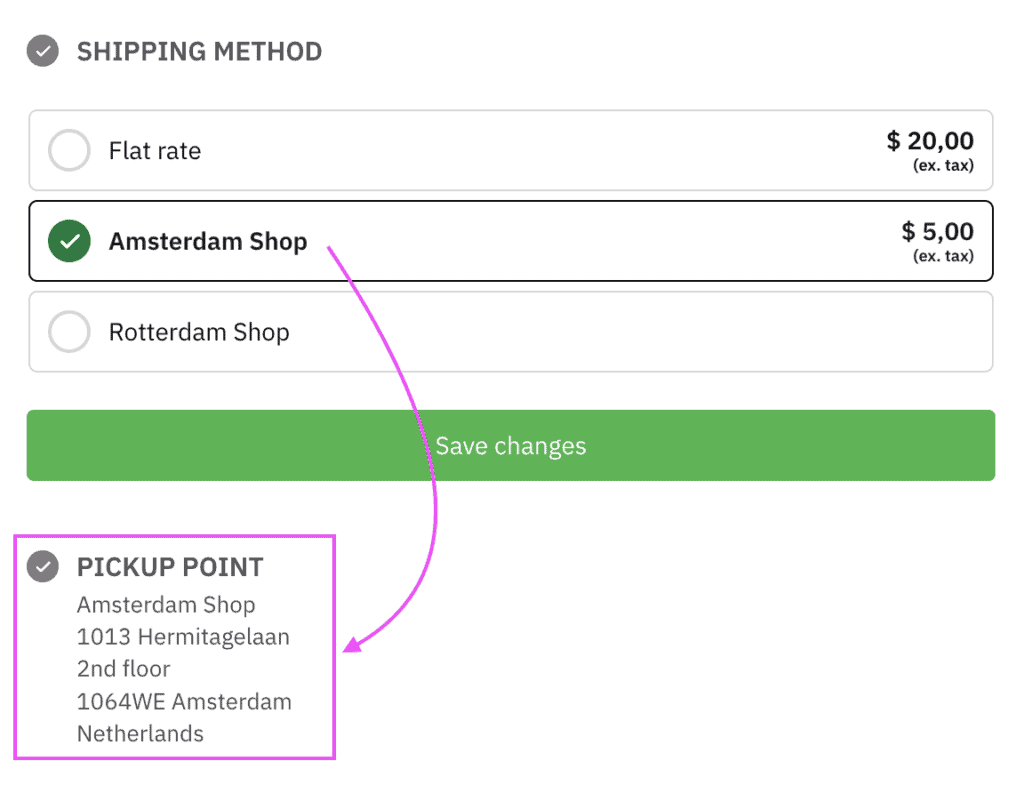
At this point, the pickup location address showed on the checkout page should change when the customer switches between the available local pickup shipping methods.
Finding the local pickup shipping method id #
In order to be able to associate each local pickup shipping method with its respective pickup location address, you will need to identify the shipping method ID for each local pickup shipping method.
Follow the instructions below to locate the shipping method ID for each local pickup shipping method that you need to customize the location address for:
- First, make sure you have added multiple local pickup shipping methods on your website settings.
- Go to the checkout page on your website.
- Fill up the first steps on the checkout page, until you reach the step with the “Shipping method” section. Usually this is the second step.
- Locate the list of shipping method options on your checkout page.
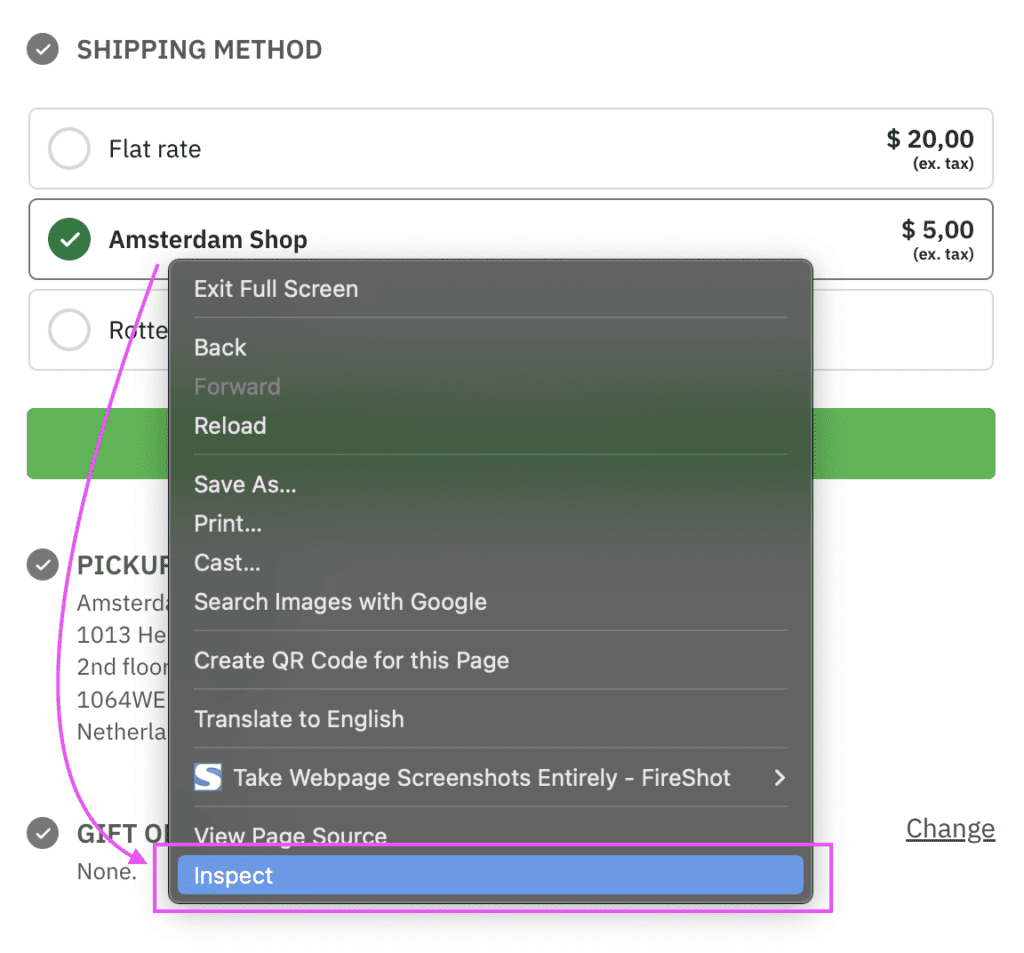
- Right-click on the shipping method you want to find the ID for, then select the option “Inspect” or “Inspect element” depending on the browser and operational system you are using.
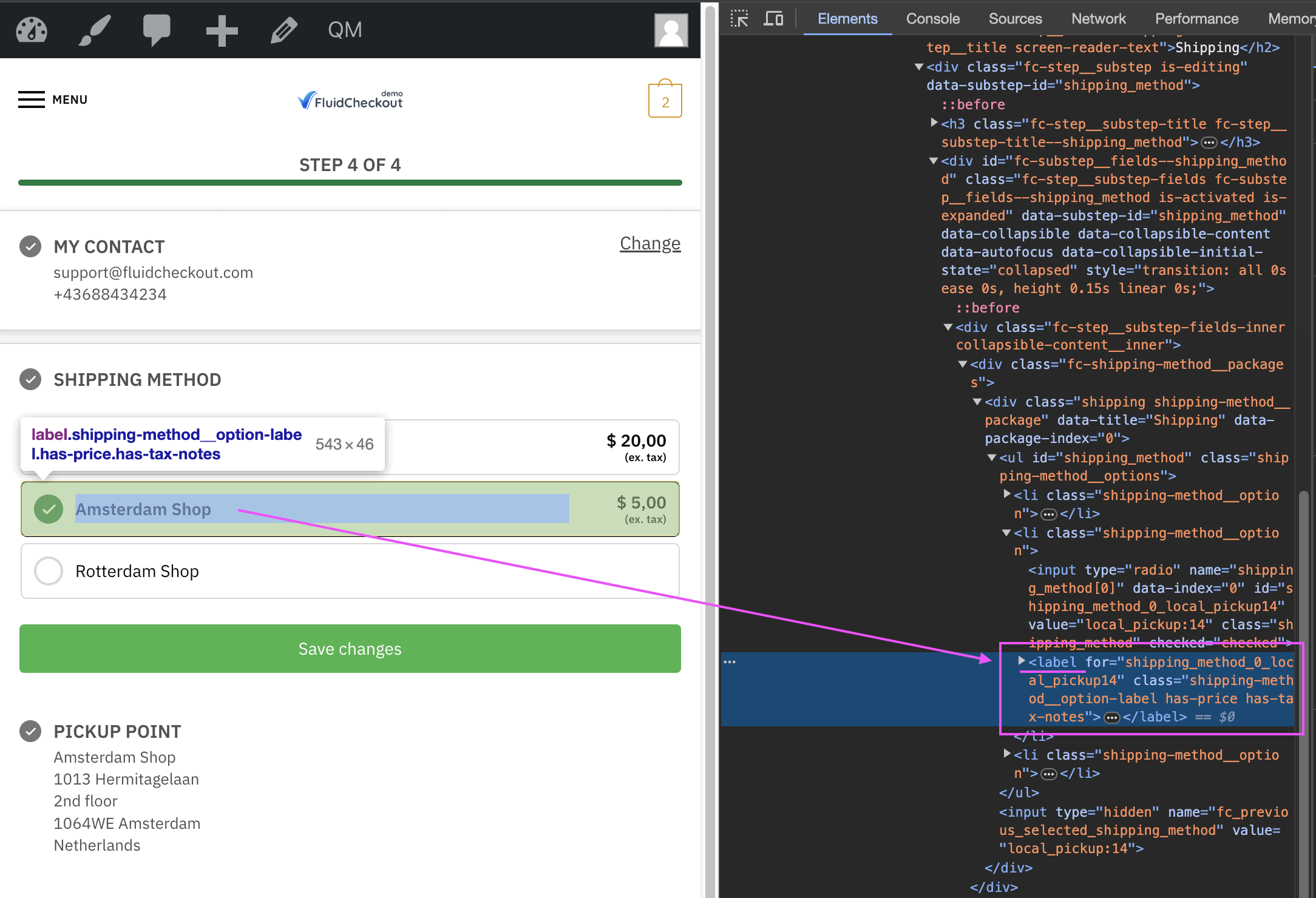
- The browser developer tools will open, automatically selecting the shipping method
label
element on the Elements tab.
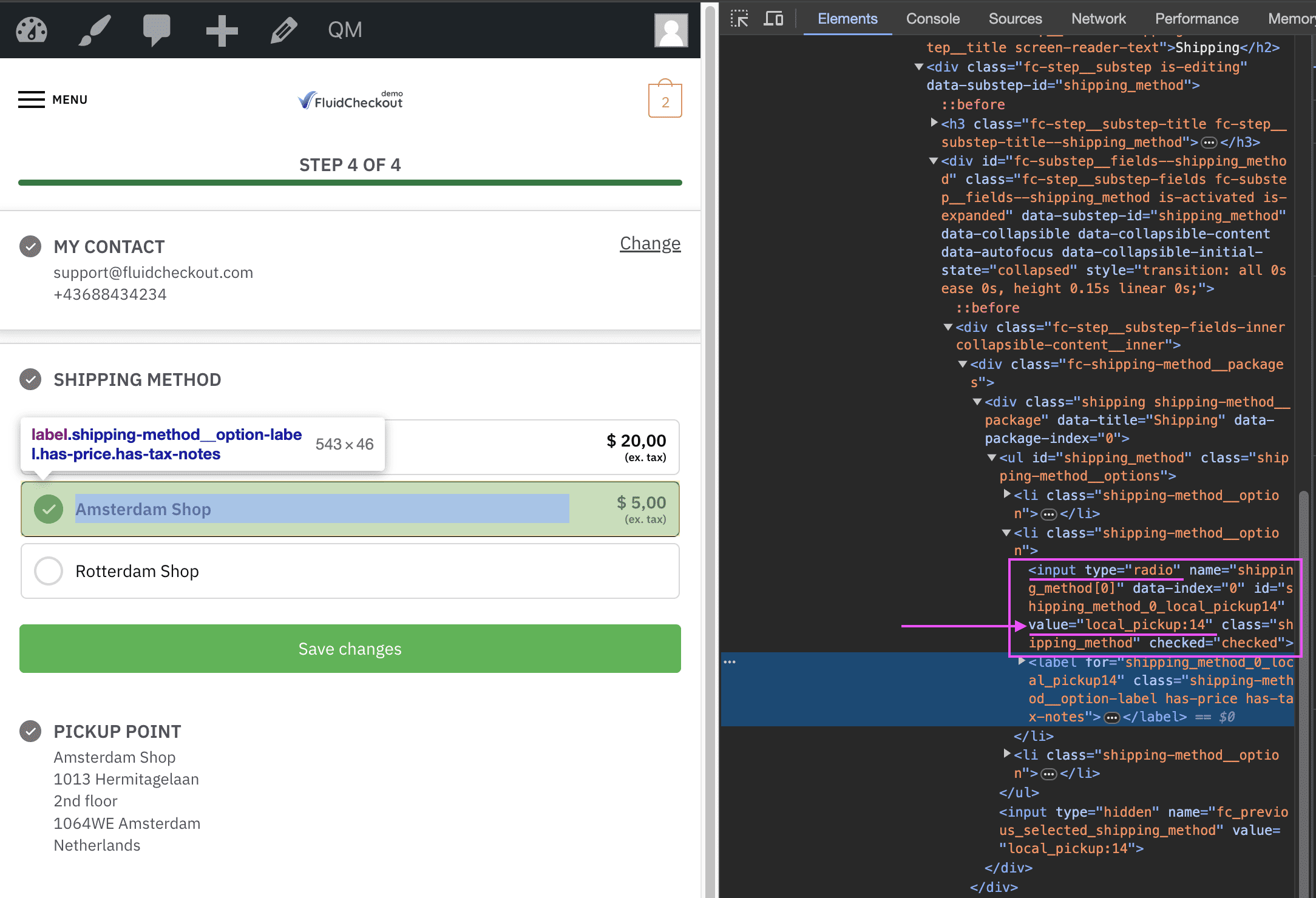
- On the browser developer tools, find the
input
element right above thelabel
element, then copy the text inside thevalue
attribute (ie.local_pickup:14
as shown on the screenshot above). - That is the shipping method ID.
- Repeat these steps for each local pickup shipping method.
You can now use those shipping method IDs to associate them with the respective pickup location address as explained in the section Customize pickup location address based on selected shipping method above.